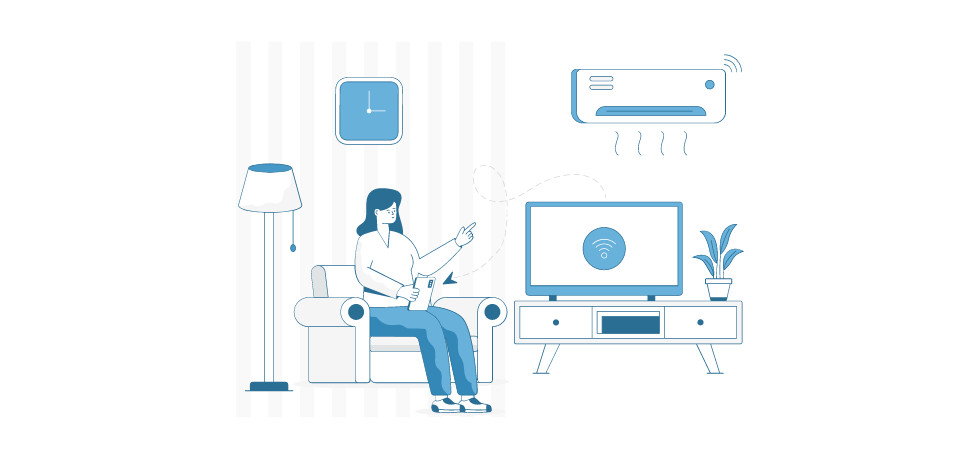
Launching a flutter app automatically at device start up is no different from launching any Android app written in either Kotlin or Java.
2-Step instructions how to launch your Flutter TV app on launch
It is really fairly simple to achieve that. There are only 2 steps involved in the entire process, you should be able to copy-paste the code below and get your app to auto-launch in 5 mins.
1. Anrdoid Manifest
First, open AnrdoidManifest.xml
located at app/src/main/AndroidManifest.xml
(if you are using Flutter) and add define the following permissions:
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" />
<uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW" />
RECEIVE_BOOT_COMPLETED
– Allows an application to receive the Intent.ACTION_BOOT_COMPLETED
which would allow you to capture the action and launch your app, keep reading.SYSTEM_ALERT_WINDOW
– Allows your application to be show on top of other apps.
Once permissions are defined, you need to add a broadcast receiver
within your <application>
tag in your manifest. Broadcast receiver simply “listens” for the boot completed
intent.
<receiver
android:enabled="true"
android:exported="true"
android:name=".BootReceiver"
android:permission="android.permission.RECEIVE_BOOT_COMPLETED">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED" />
<action android:name="android.intent.action.QUICKBOOT_POWERON" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</receiver>
2. Boot Receiver Class
The BootReceiver
will simply be called whenever the RECEIVE_BOOT_COMPLETED
is called. You can see how this is defined in your AnrdoidManifest.xml
package <your package name>
import android.content.BroadcastReceiver
import android.content.Context;
import android.content.Intent;
class BootReceiver: BroadcastReceiver() {
override fun onReceive(context: Context, intent: Intent) {
if (intent.action == Intent.ACTION_BOOT_COMPLETED) {
val i = Intent(context, MainActivity::class.java)
i.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
i.addFlags(Intent.FLAG_ACTIVITY_BROUGHT_TO_FRONT)
context.startActivity(i)
}
}
}
You need to create BootReceiver
Kotlin class located at app/src/main/kotlin/tv/<your package name>/BootReceiver.kt
(this is on the same level as your MainActivity
class. I have included a screenshot of the proper directory structure for extra clarity:
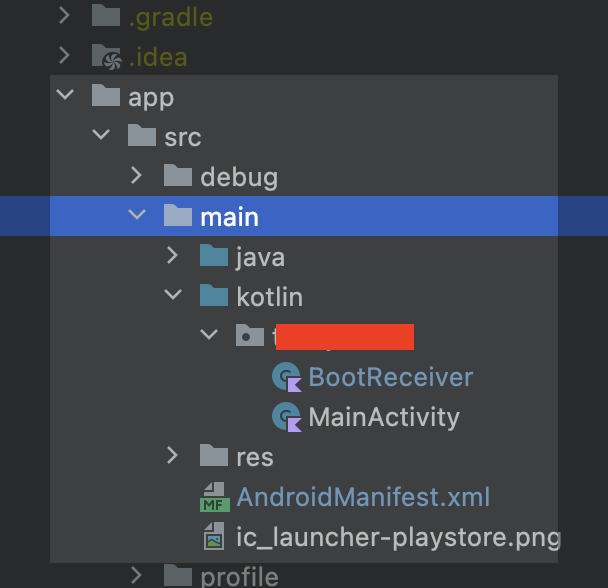
BootReceiver
class3. You are done!
Simply reboot your Android TV device and watch this app automatically launch. If you are using Flutter to deliver your app multiple platforms, which is the entire point, this approach doesn’t really affect any other platforms.
Keep in mind that this does not work in the emulator, you’d have to test this on a real device!