Create repeatable, cheap, serverless environments
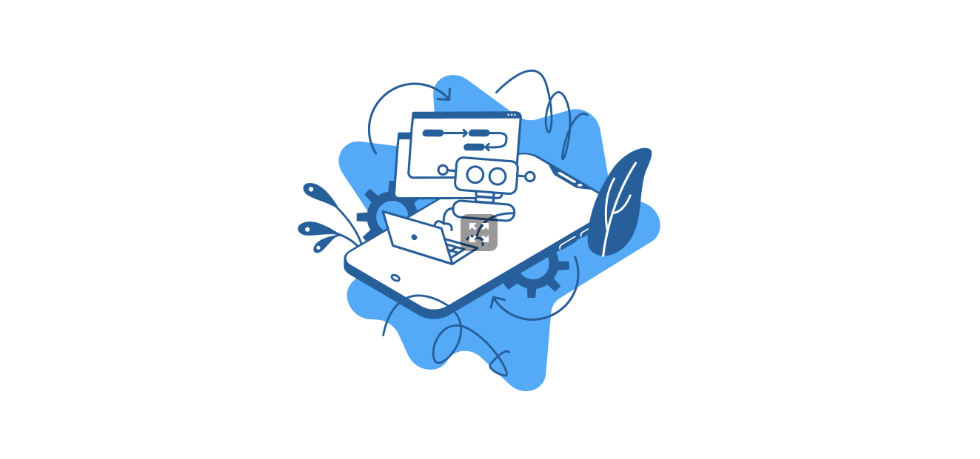
Amazon Lightsail is a cloud-based service that provides developers and businesses with an easy-to-use, low-cost solution for launching and managing virtual private servers and web applications. In addition, it offers a range of instance sizes, automatic backups, monitoring, and integration with other AWS services.
Using Ansible to provision your AWS Resources
Ansible has a half-baked community-supported module that would do most of the job for the task at hand. First, you need to make sure that you have the community.aws
module install, so just run:
ansible-galaxy collection install community.aws
To achieve this, we need 3 basic steps:
- Create a static IP: Unless you have a static IP configured, your Lightsail IP will change on every reboot, which is a massive inconvenience; a solution for this is provided below.
- Create the Lightsail instance: This step is pretty straightforward; we need to supply a few parameters further outlined in this article.
- Assign the static IP to Lightsail: After the instance and IP are created, you will have to assign the IP to the instance. There is no Ansible way to do this, so the only workaround would be to use AWS CLI in your Ansible playbook.
Now that we have our steps written out, we will turn them into ansible tasks. For this example, we will create a LAMP environment using the lamp_8_bitnami
blueprint.
We will use a {{ name }}
variable to give the instance a name, and we will prepend other names with our {{ name }}
variable for consistency. For example, as outlined above, we need to create a static IP address; part of this process is giving this resource a name, we will concatenate {{ name }}
with static_ip
in order to name the static IP resource, so our static IP resource will be named "{{ name }}_static_ip"
. We will do the same for the availability zone, namely “”{{ region }}a”`
We will use only one availability zone because we are aiming at the cheapest solution, but adding additional availability zones is definitely beneficial.
Finally, at the time of this writing, there is no way to create a static IP using Ansible directly, but that isn’t something we can’t fix with a simple bash script like so:
if [[ "{{ lightsail.instance.public_ip_address }}" != "{{ ip.static_ip.ip_address }}" ]]; then
aws lightsail attach-static-ip --static-ip-name {{ ip.static_ip.name }} --instance-name {{ name }} --region {{ region }}
fi
Putting it all together
Feel free to copy and paste this Ansible playbook into your project.
- name: Provision an Lightsail Instance.
hosts: localhost
connection: localhost
# required parameters
vars:
keypair: "{{ keypair }}"
blueprint_id: "lamp_8_bitnami"
bundle_id: "nano_2_0"
tasks:
- name: Provision a Lightsail static IP
community.aws.lightsail_static_ip:
state: present
name: "{{ name }}_static_ip"
register: "ip"
- name: Create a new Lightsail instance
community.aws.lightsail:
state: present
name: "{{ name }}"
region: "{{ region }}"
zone: "{{ region }}a"
blueprint_id: "{{ blueprint_id }}"
bundle_id: "{{ bundle_id }}"
key_pair_name: "{{ keypair }}"
register: "lightsail"
- name: Attach static IP to Lightsail instance
shell: >
if [[ "{{ lightsail.instance.public_ip_address }}" != "{{ ip.static_ip.ip_address }}" ]]; then
aws lightsail attach-static-ip --static-ip-name {{ ip.static_ip.name }} --instance-name {{ name }} --region {{ region }}
fi
Run the Ansible playbook using the following command:
ansible-playbook ./<your yaml file>.yml \ --private-key=<path to your private key>
You are done! 🚀
Don’t forget to delete all test instances that you have been creating, or AWS will keep billing you! 💸